Choosing The Right Python Task Queue
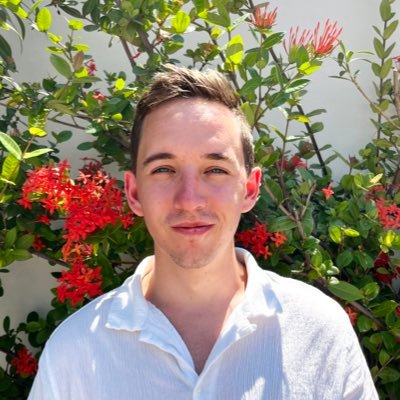
Jeff Morhous
@JeffMorhousPython task queues are an essential piece of building scalable Python apps. Whether you’re using Django, Flask, FastAPI, or something else, task queues are an essential way to offload work that could block web requests.
Choosing a task queue isn’t trival. In this article, I’ll dig into task queues, contrasting some of the best options. I’ll focus primarily on Celery and RQ, but l also touch on some lesser-known alternatives. First, let’s dig into what a task queue actually is.
Why would a Python app need a task queue?
Web apps often have tasks that either don’t come as a result of an HTTP request or take too long to handle before responding. For example, might need to generate reports, send emails, or aggregate analytics on a schedule for your Django app. Python task queues let you do this sort of thing asynchronously by pushing jobs onto a queue. You’re probably familiar with the concept of a queue whether you know it or not.
In computer science terms, queues are a data structure that organize data into a FIFO (first-in-first-out) order. Put simply, they’re lines of objects. Task queues are a handy way for your Python apps to put work into a line, where separate worker processes pick them up to execute them in the background. Offloading slow operations gives you better responsiveness for your web app, and periodic jobs let you do things on a recurring basis.
In Python, the de facto standard for years has been Celery, a complex distributed task queue. However, Celery’s complexity has led to simpler alternatives like RQ (Redis Queue). Choosing the right queue is a pretty common decision Python developers have to make, so let’s dig in.
Celery isn’t just a vegetable
Celery is a fully-featured and mature Python library for task queues. It’s a distributed task-processing system that supports multiple message brokers, flexible serialization, scheduling, and retries out of the box.
It’s well-loved in large applications and has a sizable community. However, with this power comes a learning curve and setup overhead, which we’ll get into later 👀
RQ is a fully-featured task queue
On the other hand, RQ, which stands for Redis Queue, is a much more lightweight library. It uses Redis as both the queue broker and storage, adding a bit to its simplicity. In fact, the design philosophy is simplicity, as you’ll see in its minimal API and low barrier to entry.
The trade-off is that RQ is less feature-rich than Celery. It only works with Redis (more on this later) and lacks some advanced features beyond common needs.
Comparing Celery vs RQ features
When it comes to developer experience, RQ is often praised for its simplicity. Its documentation is concise and easy to follow, reflecting the straightforward interface. On the other hand, Celery provides a bit more features out of the box.
One big difference between the two libraries is in how they handle scheduled jobs. Celery includes a simple scheduler process for periodic tasks. By itself, RQ doesn’t support scheduling tasks. There’s a separate package that provides delayed job scheduling and periodic jobs, but it involves adding and maintaining another dependency.
As you enqueue more and more background jobs, you’ll probably get an interest in having some control over task prioritization. Both Celery and RQ offer good support for prioritizing tasks, but the implementation differs a bit. Celery can route tasks to named queues, and workers can listen to multiple queues. This is super helpful when scaling, but we’ll get into that later. Setting up priority in Celery is kind of involved since you have to define separate queues for each priority and run multiple workers to consume each queue. RQ’s approach to priority is more straightforward and flexible. You can create multiple queues and RQ workers can be started to listen on more than one, in a specified order of priority.
Celery is also more flexible on where the tasks come from. Using a standard messaging protocol, Celery can be used to send tasks from languages that aren’t Python. For example, a Ruby on Rails service could enqueue a task a Celery Python worker could process it. RQ is Python-only, so you’re more locked into the Python ecosystem.
✅ Tip
Both frameworks offer a great foundation for task queueing, with Celery being more feature-rich at the cost of being more complex.
Comparing Celery vs RQ performance
If your task volume is pretty high, the choice of task queue can significantly impact performance. Celery, especially when paired with a fast broker like Redis, can perform quite well.
RQ may not be quite as fast. In one benchmark of 20,000 small jobs with 10 workers, RQ took 51 seconds to complete, whereas Celery (using threads) took 12 seconds.
Celery’s implementation seems to scale better for large loads. Newer versions of RQ have introduced a worker pool and other optimizations, but the performance difference is still dramatic.
How do Celery and RQ handle reliability and task failure?
One thing that concerns developers when working with task queues is ensuring no tasks are lost. Here, the choice of backend broker matters a lot. Celery supports brokers like RabbitMQ, which offers durable message delivery, meaning a crashed worker won’t lose a task. RQ is tied to Redis (hence the ‘R’ 😉), which does not guarantee the same level of durability or reliability as a message queue like RabbitMQ.
If an RQ worker process crashes after grabbing a task from Redis to work, that task might be lost. In short, Celery with the right message broker can achieve better reliability than RQ with Redis alone.
It’s also possible that tasks can fail, which is something that your task queue should be prepared for. Both Celery and RQ support retrying tasks on failure. With Celery, a task can be configured with a max retry count and interval, and you can even call self.retry()
in task code to re-queue it after an exception occurs. Celery also has support for exponential backoff. If an RQ task fails, RQ will enqueue a new attempt according to the policy you configured up-front.
What does operating Celery and RQ in production look like?
If you’re the developer who actually has to support an application with task queues, you’re probably interested in how these systems run in production and integrate with your infrastructure. I can’t tell you how many times I’ve been paged in the middle of the night as a result of a scheduled task.
Celery typically requires running a separate message broker service like RabbitMQ or Redis. We’ll get into choosing this in the next section, but you should know that operating RabbitMQ is a lot more involved than Redis. If you’re not using a broker already, adopting RabbitMQ just for Celery adds operational overhead that probably isn’t preferable. Redis is simpler to run in my opinion, and many teams already have Redis for caching. RQ’s big operational advantage is that you don’t need to run any new service aside from Redis.
Both Celery and RQ can run just fine in Docker. A Celery worker is just a process that you can containerize, and an RQ worker is similarly containerizable.
✅ Tip
In short, RQ is pretty easy to deploy and maintain, especially if you already have Redis. Celery requires more setup, but it might be more appropriate at scale. Are you seeing a theme yet?
Scaling Python task queues
Both Celery and RQ are easily scaled horizontally and vertically to handle huge amounts of work. You scale each horizontally by adding more workers and vertically by increasing the resources each worker has. When scaling horizontally, the goal is to avoid queue backups, and we can quantify that in terms of task queue latency. Think, “How long tasks are sitting in the queue before being picked up for processing?” This is the metric you want to keep in mind when determining if you need to add or remove workers.
What’s an acceptable queue latency? That’s up to you! You’ll need as many workers as necessary to avoid exceeding your SLO (service level objective). Figuring out that magic number of workers is nearly impossible because your workload is constantly changing, so whenever possible you should automate your horizontal scaling with an autoscaler like Judoscale. Judoscale integrates quickly with all the major platforms, and is easy to configure with Django, Flask, and FastAPI.
Whichever autoscaler you end up choosing, just make sure you’re scaling based on queue latency rather than compute metrics like CPU. CPU is a pretty poor metric for autoscaling. Task queues can easily back up without a CPU spike, leaving you in the dark. Judoscale autoscales based on queue latency by default, and it works great with both Celery and RQ (and it’ll also autoscale your web services for Django, Flask, and FastAPI!).
Exploring some less popular options for task queues
Apart from Celery and RQ, the Python ecosystem has other (less popular) task queues that you might be interested in.
Huey for task queues
Huey is a lightweight Python task queue that is very lightweight in terms of code, setup, and resources. It still provides the expected core features and is typically backed by Redis. It is designed for small-to-medium apps that need background jobs but not the full feature set (and complexity 🥲) of Celery.
Dramatiq for task queues
Dramatiq is another task queue library focused on simplicity, reliability, and performance. Dramatiq supports both RabbitMQ and Redis as brokers. It’s similar to Celery, but intentionally has a smaller feature set than Celery. There’s no built-in scheduler, but nothing’s stopping you from adding one. Some consider Dramatiq a more modern Celery alternative for brand-new projects when you don’t need Celery’s entire feature set but want better performance out of the gate.
Choosing between task queue backend brokers
When you use a background job queue, you’re essentially pushing tasks into a shared system where workers can come pick them up later. That shared place is called the backend broker. The broker you choose stores the tasks and sits between your app and your workers. If a task has been enqueued but not picked up by a worker, it’s in the broker.
Developers that use Celery and those that use RQ commonly use Redis as the backend broker, but it’s not the only option. Celery’s documentation points out some options, including RabbitMQ and Amazon SQS. Each comes with trade-offs in terms of reliability, throughput, and operational complexity.
If you’re already running Redis for caching or sessions, using it as a task queue broker is a common choice. RabbitMQ, on the other hand, excels when you need delivery guarantees or you’re working in a system that has more than one language (yay, microservices!). If you’re deploying on cloud platforms, you might also consider managed brokers like Amazon SQS.
✅ Tip
Use Redis if you want simplicity. Consider RabbitMQ if you need performance stronger guarantees. And if you’re cloud-native and don’t want to manage a broker at all, SQS could be a good option.
So which task queue should you choose?
Since there’s so many good options for Python task queues, choosing can be a challenge. Even if you’ve narrowed it down to Celery vs RQ, it’s not an easy decision.
RQ is likely the most simple approach to task queueing you could choose. In my opinion, it’s the ideal choice for most apps, especially if it can handle the volume of tasks your app needs. If you already use Redis, RQ is an even more straightforward choice.
Celery brings even more features and tosses in some better scalability. If you’re enqueuing tasks at massive scale, it’s probably the better choice. The tradeoff of choosing Celery over RQ is the additional complexity of operating and learning it, but many find that cost justified in the long run.
Other alternatives like Dramatiq and Huey are neat but don’t show enough of a difference from the more popular options that they seem attractive to me. If I had to choose today, I’d pick RQ until it didn’t work for me anymore, then migrate to Celery.