Scaling Python Task Queues Effectively
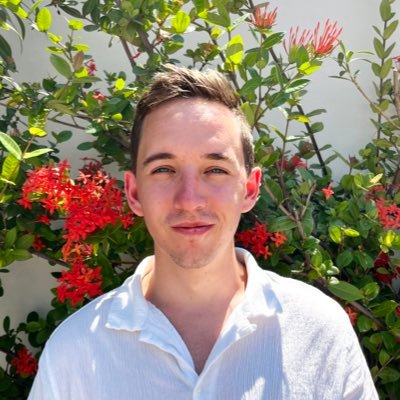
Jeff Morhous
@JeffMorhousScaling your Python task queues can mean the difference between snappy user experiences and “why hasn’t this email arrived yet?” support tickets. When traffic spikes or your Python app starts doing intensive work in the background, tasks can pile up, queue latency climbs, and users start having problems.
Whether you’re drowning in tasks or spending too much on overprovisioned workers, you’re in the right place.
Why scaling a task queue matters for you
If your Python app is small, a single worker process handling your task queue might be enough. But as your web app grows, the volume of tasks often increases with it. Whether you’re using task queues to send emails or process data, if your task queue can’t keep up, jobs will start backing up. High queue latency (tasks waiting too long to run) can be problematic.
Queue latency is a crucial metric for performance. A task taking a long time from enqueue to successful completion translates directly to slower feedback for users. For example, if an email task sits in a queue for 25 minutes before actually sending, users won’t be happy (trust me, I’ve been there 🤦♂️).
Without proper scaling, your queue will eventually stop processing jobs fast enough for your standards. Proper scaling ensures you have enough worker power to handle traffic bursts. For instance, if a marketing campaign triggers thousands of jobs per minute, scaling out workers will let you chew through them faster and avoid a massive backlog.
There are a number of levers you can pull that affect scaling, starting with the task queue’s message broker. Let’s take a look into why that matters.
How a message broker choice comes into play
Your task queue’s message broker is the intermediary system that holds queued tasks. Celery supports multiple brokers including Redis and RabbitMQ, while RQ is designed to use Redis exclusively. While choosing a Python task queue is obviously high-stakes, the message broker you choose also has a surprising impact on how well your system scales under load.
Take a look at the earlier diagram, this time with the message broker, worker process, and application process all pointed out:
Redis is a popular message broker choice for both Celery and RQ. If you’re not already familiar, Redis is an in-memory data store known for speed and high throughput. It’s commonly used for caching, but its speed makes it a good choice for task queues. It’s simple to set up and operate, and if you’re already using it for caching in your app, adding it as a Celery/RQ broker is straightforward.
RabbitMQ is a dedicated message broker, and it’s a popular Celery broker choice. Still, it’s unavailable if you’re using RQ. RabbitMQ stands out for its reliability and flexibility. It provides durable message queues and acknowledgments out of the box which makes it a more fully-featured choice. If a Celery worker crashes while processing a task, RabbitMQ will notice the unacknowledged message and enqueue the task again, ensuring no task is lost. The tradeoff is that it’s heavier to operate than Redis and can have higher latency than Redis for simple queues.
My choice is likely to be Redis because of its simplicity and raw speed, but RabbitMQ might be better if you absolutely need stronger guarantees out of the box.
Scaling worker processes
The most common way to effectively scale a Python task queue is by increasing your worker concurrency. There are two main approaches to processing more tasks in parallel: vertical scaling (using “bigger” workers with more threads/processes) and horizontal scaling (increasing quantities of worker processes or machines). You’ll likely want to use some combination of both scaling approaches.
Vertical scaling worker processes
As we mentioned above, vertical scaling means increasing the capacity of each worker process with additional resources. This is tricky for Python task queues.
Celery can be both multi-threaded and multi-process, making it possible to take advantage of of more power. With an appropriate amount of resources, you can raise the concurrency in Celery by using more child processes and/or threads for each worker process.
By contrast, RQ is single-threaded and single-process. If you’re using RQ, one process can handle just one task, but running RQ on a machine with more CPU/RAM is still a somewhat useful vertical scaling of that worker.
Whether you’re using RQ or Celery, vertical scaling by adding more RAM can help a worker get through particularly memory-hungry tasks.
Horizontal scaling worker processes
Scaling horizontally means adding more worker processes or machines to consume tasks at once. Celery and RQ both support this without too much hassle. You can have several workers connecting to the same broker queue, each pulling and working their own tasks.
With Celery, each worker instance can process tasks concurrently according to their concurrency setting. For RQ, you simply run more worker instances, each locked to a single task at a time. The message broker, since it’s distributed, will distribute tasks to each worker process. Horizontal scaling is my favorite way to respond to increasing task queue demands, especially if you autoscale your workers.
It’s worth noting that if your workers are bumping up against resource limits as they process a given task, horizontal scaling won’t be all that effective. Giving your worker processes enough resources (whether by adding RAM or staying single-threaded) ensures that adding more workers actually helps with throughput.
Autoscaling workers based on queue latency
Manually deciding when to add more or remove workers would be painful! Most Django or Flask apps have variable workloads and traffic fluctuates. Autoscaling isn’t just for web apps, it’s a great way to make sure you’re always running an appropriate amount of workers for your task queues.
So what metric should drive autoscaling for task queues? Many autoscaling solutions use CPU usage as a proxy for worker process stress, but it’s a poor indicator of background job load. Your workers can be mostly idle on CPU yet you still have a growing queue. A better metric for scaling task queues is queue latency, which measures how long tasks actually wait before being processed.
A grocery store example
Imagine a grocery store with a queue of customers (tasks) and two checkout clerks (worker threads). Both clerks might be helping a customer, but they might be waiting on a credit card machine (I/O) instead of doing actual work. The CPU usage might be low, but there’s still a growing queue of angry customers (aging tasks).
Looking at how fast the hands are moving on the cashier might not tell you if you need to hire more cashiers, but a long line of irritated customers is a strong signal.
Simplifying autoscaling based on queue latency
Tools like Judoscale simplify this for you with language-specific libraries (including Python!) that report queue metrics and handle the decision logic for you. Responsive task queues aren’t the only benefit, you’ll also save money by avoiding paying for more workers than needed.
Even with autoscaling, there are a few things you can do to make sure you’re using your worker processes effectively, starting with fanning out large jobs.
Fanning out large tasks
One tried and true method for scaling background tasks is to fan out large tasks so each piece takes overall less time to complete.
For example, you might have a background task that iterates through all the events
objects in your database and sends a reminder for each one that occurs in the next 24 hours. If you have a lot of events in your database, this doesn’t scale well!
You could instead split this into two separate tasks. One task could iterate through all the events and enqueue a different task that sends a reminder for a given event.
Not only does this strategy break up long-running tasks, but it makes it handle task failure better. With one long-running task, any problem requires you to run the whole job again. By fanning this sort of task out into smaller tasks, an individual failure is much more isolated.
Task isolation
If you have specific tasks that are long-running or use lots of memory, this can wreak havoc with other tasks on the same queues or the same workers. At scale, you want to avoid these sorts of greedy tasks starving out tasks that can be completed quickly. The best approach here is to isolate those tasks to their own queues and worker processes.
One good way to accomplish this is to separate tasks by their target time to completion (or SLA). Then, you can enqueue these different tasks into different queues, even naming the queues based on the SLA. Tasks that should be completed in 5 seconds can be put into a five_second
queue, and an independent worker can be assigned to each queue.
With this setup, those “trouble-maker” jobs can only impact other troublemakers like them, avoiding slowing down queue latency for otherwise quick tasks.
Best practices for scaling Celery
Celery is a mature framework with lots of configuration options, which means there are plenty of options to tune it to your needs.
Celery’s default concurrency is the number of CPUs on the machine. That’s a good starting point, but don’t be afraid to experiment. Use a good APM and monitor your Celery workers’ CPU and memory consumption.
The Celery documentation points out that beyond a certain point, adding more processes for every worker can hurt performance, and multiple worker instances might perform better. You can also use the built-in process autoscaling to see if it fits your needs.
Celery’s default prefetch multiplier is 4, meaning each worker process will reserve 4 tasks at a time from the broker. One process might grab 4 tasks, working on the first one while 3 sit waiting within that worker, even if other workers are idle. If you have many long-running tasks, try setting worker_prefetch_multiplier = 1
so that a worker only takes one task at a time.
Best practices for scaling RQ
Redis Queue (RQ) is intentionally simpler than Celery, so you have fewer RQ-specific options for scaling. One of RQ’s most popular attributes is that it’s _ust Python + Redis. You can hop into a Python shell, query Redis for queue details, inspect tasks, etc.
As you scale, build some simple monitoring around RQ. Consider a small dashboard that shows queue sizes, or even just use the built-in RQ monitoring to get a high-level view of your workers and queues. Simplicity also means fewer failure modes: you don’t have a complex cluster of components, just many processes doing the same thing. This can be an advantage for autoscaling!
Strategize your scaling
Scaling your Python task queues isn’t optional. With the right broker, properly tuned worker processes, and queue latency-driven autoscaling, you can build a background system that feels invisible to users.
If you combine the nailing fundamentals with a good autoscaling setup, you and your users will be grateful.